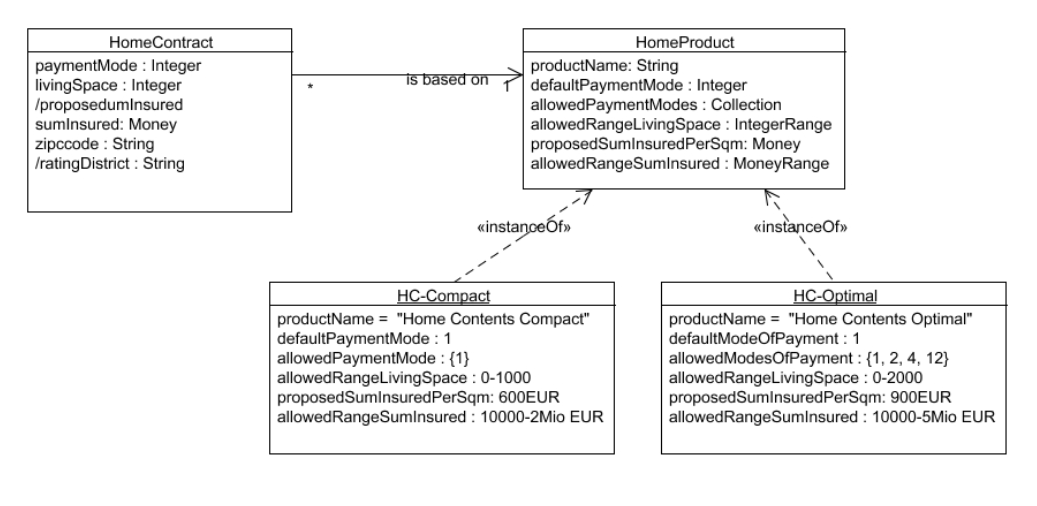
Part 1: Modeling and Product Configuration
Adding Product Aspects to the Model
Now we will finally start to model the product aspects. Before we do this with Faktor-IPS, we will discuss the design at the model level.
Let us have a look at the properties defined for our HomeContract
class so far and consider which aspects of these properties should be configurable in an insurance product:
Properties of HomeContract | Configuration options |
---|---|
|
The payment modes permitted in the contract. |
|
The range (min, max) of the living space. |
|
Definition of a default value per square meter of living space. The proposed sum insured will then be computed by multiplying this value by the living space [9]. |
|
The value range of the sum insured. |
[9] Alternatively, we could implement this configuration using a formula to compute a proposed sum insured. But we will focus on the factor for a start.
We will create two home contents products. HC-Optimal
will offer a comprehensive insurance coverage, while HC-Compact
provides a basic insurance at low cost. The following table shows the properties of both products with respect to the above configuration possibilities:
Configuration Option | HC-Compact | HC-Optimal |
---|---|---|
Default paymentMode |
annually |
annually |
Allowed paymentMode |
bi-annually, annually |
monthly, quarterly, bi-annually, annually |
Allowed range of living space |
0-1000 sqm |
0-2000 sqm |
Proposed sum insured per square meter of living space |
600 Euro |
900 Euro |
Sum insured |
10 Tsd - 2 Mio Euro |
10 Tsd - 5 Mio Euro |
We represent this in the model by introducing a class named HomeProduct
. This product contains all properties and configuration possibilities that have to be identical for home contracts based on the same product. Both HC-Optimal
and HC-Compact
are instances of the HomeProduct
class. The model is shown in the following UML diagram:
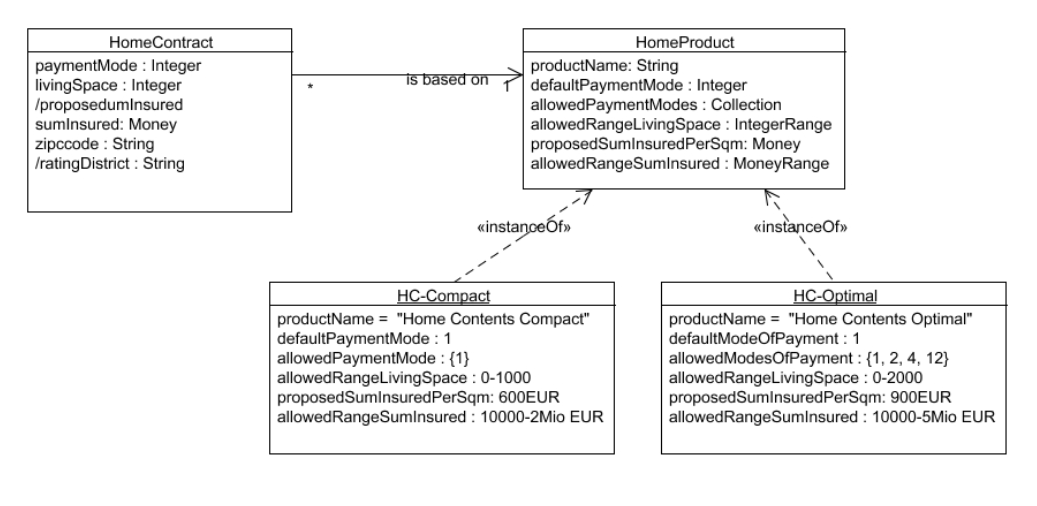
Let us now add product classes to our model in Faktor-IPS. First, we will define the home.HomeProduct
class. To do this, click the toolbar button . When the wizard opens, enter the name of the new class ("HomeProduct") and, in the Policy Component Type field, enter "home.HomeContract". When you click Finish, the editor for product classes will open for you.
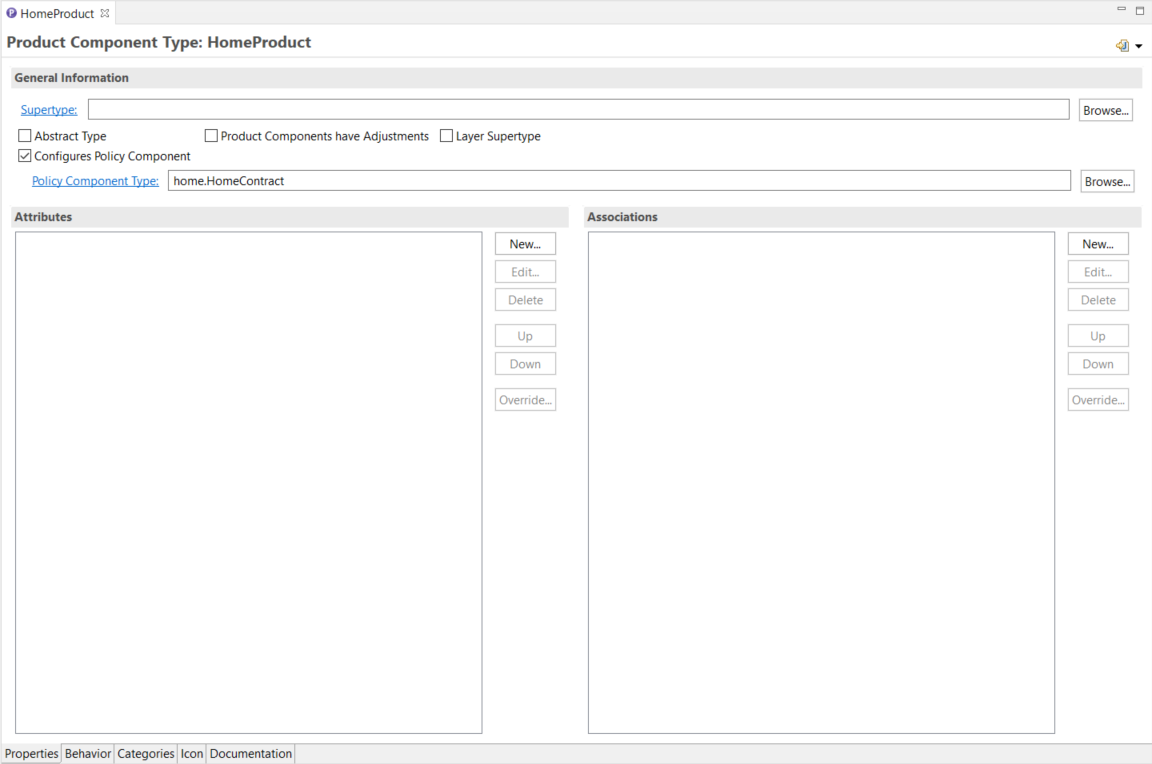
In the General Information section we can see that the HomeProduct
class configures the HomeContract
class.
This corresponds to the information we provided before. Otherwise the first editor page is structured similarly to the contract class editor [10].
[10] Within Preferences you can choose if you want to get all information about a given class on one page or on two pages.
At the same time, Faktor-IPS has generated the implementation class "HomeProduct".
- The following aspects should be configurable in the HomeProduct class
-
-
the product name
-
the legal paymentModes as well as the default paymentMode
-
Let us start with the product name. Create a new String attribute named productname
, just like you would create a contract class attribute. As with contract class attributes, the legal values can be limited using ranges or enumerations, but we will not use this option for our product names. The dialog box is shown in the following figure.
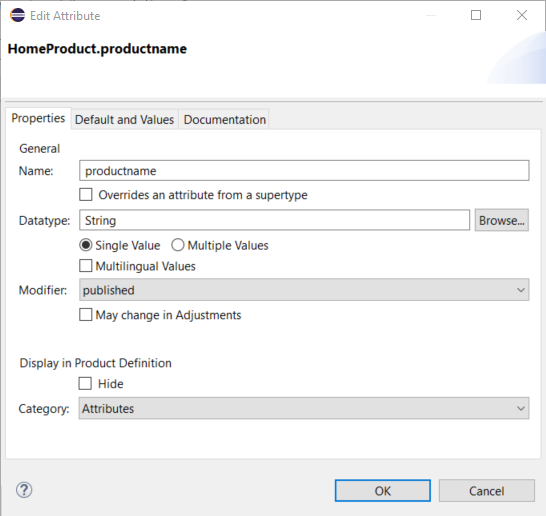
Now, we will define that the allowable modes of payment for a HomeContract and the default payment mode can be configured within the product. To do this, we will first open the editor for the HomeContract
class. In the General Information section, the wizard has stated that the HomeContract class is configurable by the HomeProduct
class.
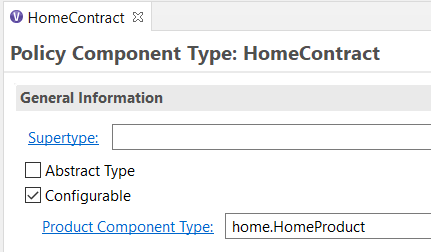
Next, open the dialog box for editing the paymentMode
attribute. Since contracts are now declared as configurable, the dialog box includes a new Configuration section. In this section you can determine whether and how each attribute can be configured. Depending on the attribute type, there are various ways to do so.
To be able to define the valid payment modes and the default payment mode within the product, you have to mark the appropriate checkbox. Now close the dialog box and save your settings.
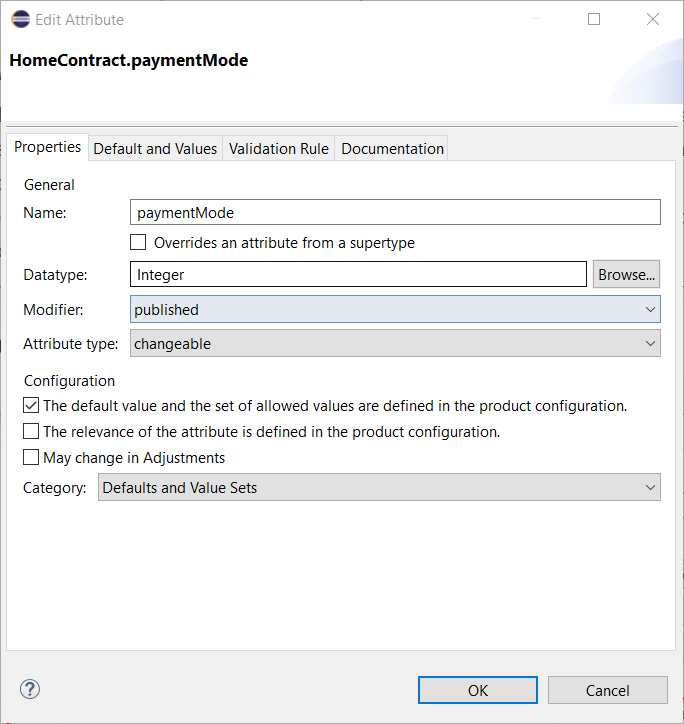
Note that the checkbox for "May change in Adjustments" is not checked.
Let us now have a look at the source code. The class HomeProduct
now contains methods to retrieve the product name, the default payment mode, and the allowable values for payment mode, respectively.
/**
* Returns the value of productname.
*
* @generated
*/
@IpsAttribute(name = "productname", kind = AttributeKind.CONSTANT, valueSetKind = ValueSetKind.AllValues)
@IpsGenerated
public String getProductname() {
return productname;
}
/**
* Returns the default value for paymentMode.
*
* @generated
*/
@IpsDefaultValue("paymentMode")
@IpsGenerated
public Integer getDefaultValuePaymentMode() {
return defaultValuePaymentMode;
}
/**
* Returns the set of allowed values for the property paymentMode.
*
* @generated
*/
@IpsAllowedValues("paymentMode")
@IpsGenerated
public OrderedValueSet<Integer> getAllowedValuesForPaymentMode(IValidationContext context) {
return allowedValuesForPaymentMode;
}
In the class HomeContract
exist methods to access the HomeProduct
.
/**
* Returns the HomeProduct that configures this object.
*
* @generated
*/
@IpsGenerated
public HomeProduct getHomeProduct() {
return (HomeProduct) getProductComponent();
}
/**
* Sets the new HomeProduct that configures this object.
*
* @param homeProduct The new HomeProduct.
* @param initPropertiesWithConfiguratedDefaults <code>true</code> if the
* properties should be
* initialized with the defaults
* defined in the HomeProduct.
*
* @generated
*/
@IpsGenerated
public void setHomeProduct(HomeProduct homeProduct, boolean initPropertiesWithConfiguratedDefaults) {
setProductComponent(homeProduct);
if (initPropertiesWithConfiguratedDefaults) {
initialize();
}
}
Our last step is to mark the attributes livingSpace
and sumInsured
as configurable, as we did it before with paymentMode
.
Next, we will review our computation of the proposed sum insured. In Extending the Home Contens Model, we implemented the getProposedSumInsured()
method of the HomeContract
class like this:
/**
* Returns the proposedSumInsured.
*
* @restrainedmodifiable
*/
@IpsAttribute(name = "proposedSumInsured", kind = AttributeKind.DERIVED_ON_THE_FLY, valueSetKind = ValueSetKind.AllValues)
@IpsGenerated
public Money getProposedSumInsured() {
// begin-user-code
// TODO: later we'll implement this with a product data lookup
return Money.euro(650).multiply(livingSpace);
// end-user-code
}
As a next step, we want to be able to configure the multiplier for the home product. To do this, we first add a new attribute named proposedSumInsuredPerSqm
of the type Money to the HomeProduct
class. This is the suggested value per square meter of living space.
After saving the HomeProduct class, Faktor-IPS has generated the appropriate getter method getProposedSumInsuredPerSqm()
to the HomeProduct
class. We will now take advantage of this getter to compute our proposal for the sum insured.
Customize the source code in the HomeContract
class as follows:
/**
* Returns the proposedSumInsured.
*
* @restrainedmodifiable
*/
@IpsAttribute(name = "proposedSumInsured", kind = AttributeKind.DERIVED_ON_THE_FLY, valueSetKind = ValueSetKind.AllValues)
@IpsGenerated
public Money getProposedSumInsured() {
// begin-user-code
HomeProduct prod= getHomeProduct();
if(prod==null) {
return Money.NULL;
}
return prod.getProposedSumInsuredPerSqm().multiply(livingSpace);
// end-user-code
}
Let us now define the "product side" of our model for the base coverage. To do this we mark the class HomeBaseCoverage
as "configurable". Our new class for this purpose will be named HomeBaseCoverageType
. For this class, you define an attribute named "name" of type String. In the course of this tutorial, we will further extend this class.
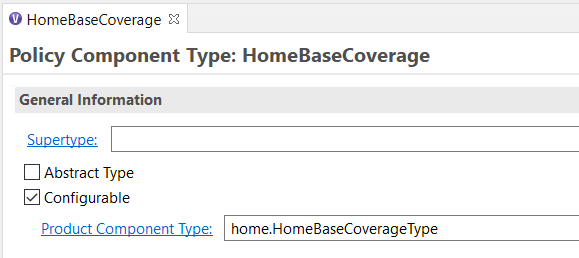
At the end of this chapter, we will consider the relationships between the classes on the product side. By means of these relationships, we want to capture which (home) coverage types are included in which (home) products. The model is depicted in the following UML diagram:
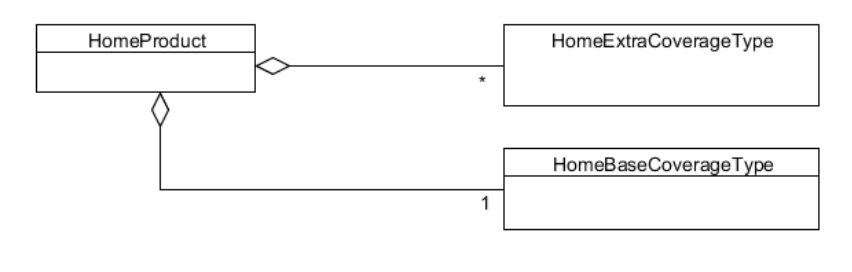
The home product uses precisely one base coverage type and any number of extra coverage types. Conversely, one base coverage type or one extra coverage type can apply to any number of home products. The primary navigation always goes from home product to coverage type (base or extra), but not in the other direction, because a coverage type should never depend on the products that use it.
Finally, let us define the relationship between HomeProduct
and HomeBaseCoveragesType
in Faktor-IPS. To do this, open the editor for the HomeProduct
class and click _ New_ in the Associations section to create a new relationship. The following dialog box will open for you; please insert the same values as shown in the figure. Note that you must set the maximum cardinality to one. The extra coverage type will be created in part 2 of the tutorial.
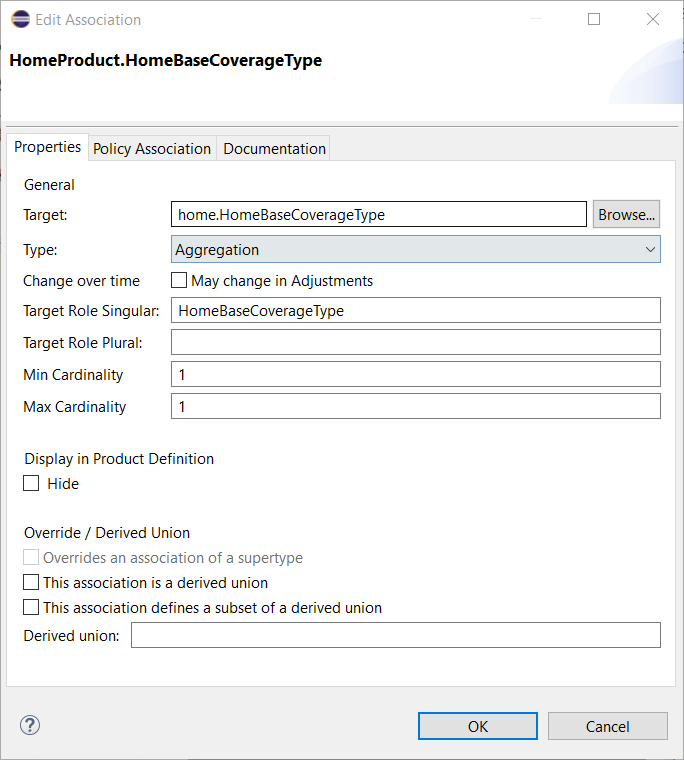