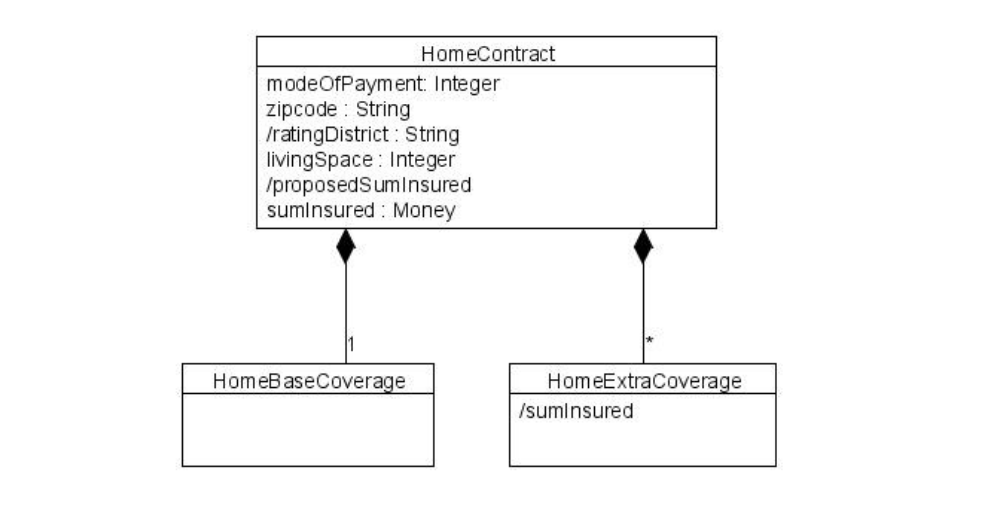
Part 1: Modeling and Product Configuration
Extending the Home Contents Model
In this section we will expand our home contents model. The following figure shows the model as is.
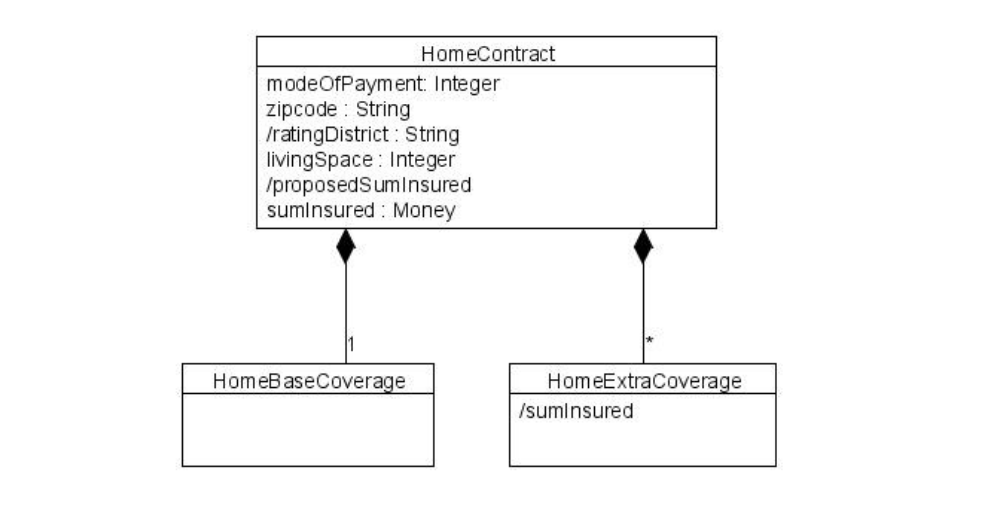
Each HomeContract
must include precisely one base coverage and any number of extra coverages. The base coverage always covers the sum insured according to the contract. In addition, a HomeContract
can include optional extra coverages, typically covering risks like bicycle theft or overvoltage damage. We will cover these in the second part of our tutorial and focus on the HomeContract
and HomeBaseCoverage
for the time being.
Let us open the HomeContract
class and define its attributes:
Name: Datatype | Description, Comments |
---|---|
|
The zipcode of the insured home contents |
|
The rating district (I, II, III, IV, or V) depends on the zipcode
and determines the insurance rate. |
|
The living space of the insured home contents in square meters. Allowable values range from min=0 to unlimited. The value range is defined on the second dialog page. If you want the value range to be unlimited, leave the maximum field empty. |
|
A suggested value for the sum insured. It is determined based
on the living space. |
|
The sum insured. |
The editor showing the HomeContract
class should now look as follows:
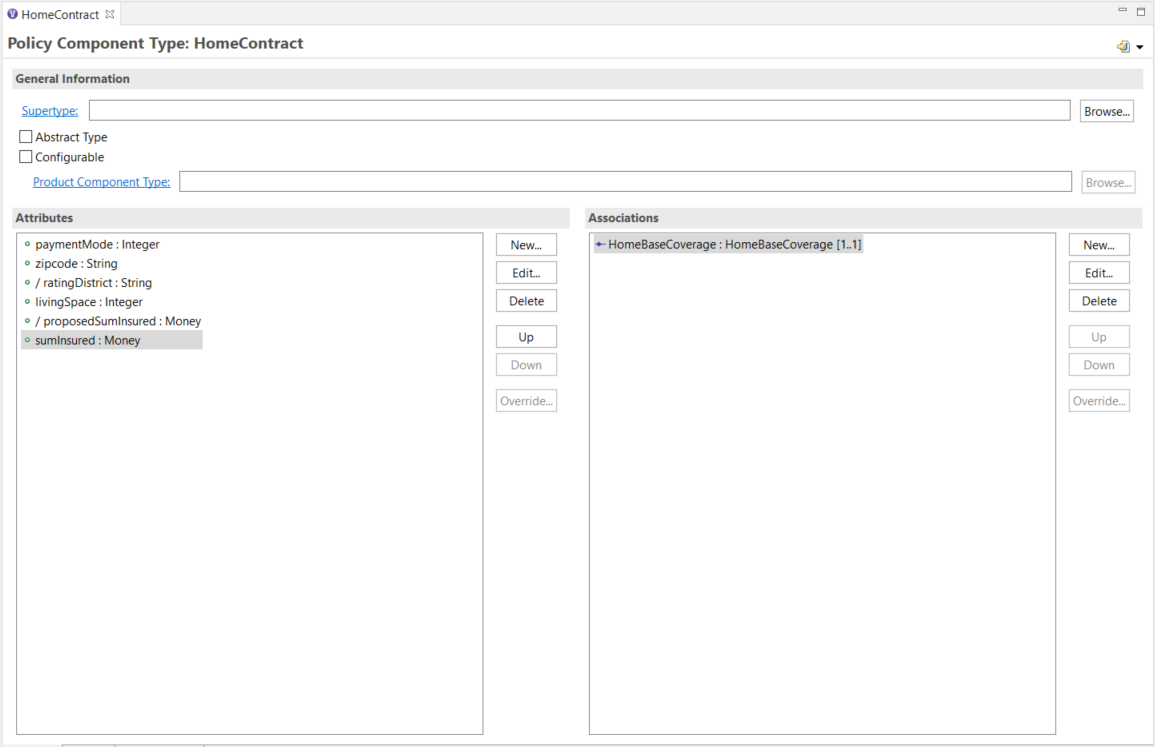
The derived attributes are prefixed with a slash according to the UML notation.
Next, open the HomeContract
class in the Java Editor and implement the getter methods for the derived attributes "ratingDistrict" and "proposedSumInsured" as follows.
/**
* Returns the ratingDistrict.
*
* @restrainedmodifiable
*/
@IpsAttribute(name = "ratingDistrict", kind = AttributeKind.DERIVED_ON_THE_FLY, valueSetKind = ValueSetKind.AllValues)
@IpsGenerated
public String getRatingDistrict() {
// begin-user-code
// TODO: later we'll implement this with a table lookup
return "I";
// end-user-code
}
/**
* Returns the proposedSumInsured.
*
* @restrainedmodifiable
*/
@IpsAttribute(name = "proposedSumInsured", kind = AttributeKind.DERIVED_ON_THE_FLY, valueSetKind = ValueSetKind.AllValues)
@IpsGenerated
public Money getProposedSumInsured() {
// begin-user-code
// TODO: later we'll implement this with a product data lookup
return Money.euro(650).multiply(livingSpace);
// end-user-code
}
@restrainedmodifiable
is generated by the generator instead of @generated
for certain methods (e.g. in generated test classes or rules) and indicates that the developer can add their own code.
The section in which the own code may appear is indicated by comments.@restrainedmodifiable
can only be used if the annotation was created by the generator.
A replacement of @generated
and insertion of the appropriate comment lines does not work and is overwritten by the generator.