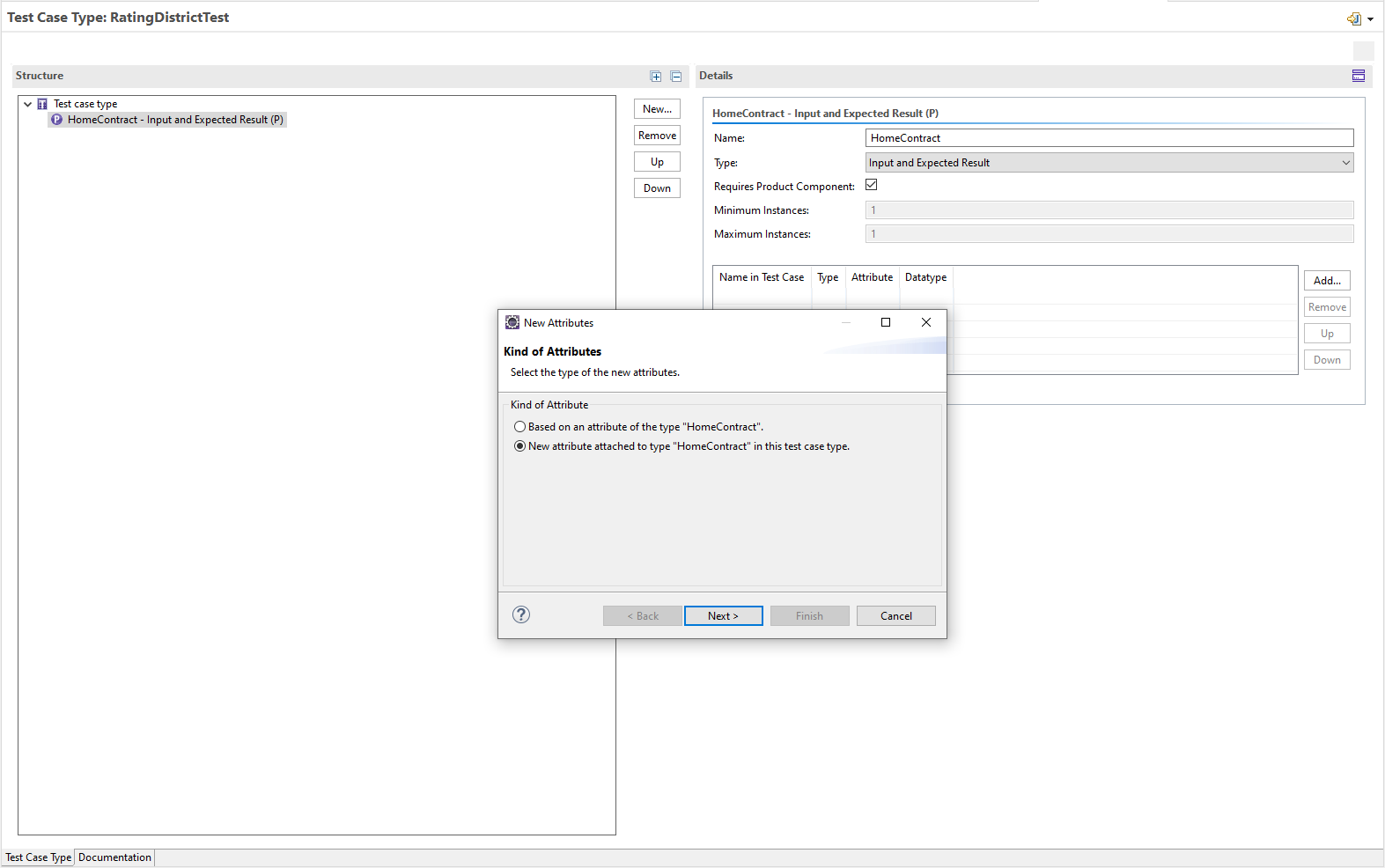
Part 3: Testing with Faktor-IPS
Special case: Testing Derived Attributes
So far, we have implemented the comparison logic in the test case type based on the comparison of member variables of the test objects. In the Input instance, we had the attribute computed, while in the Expected instance, we entered it into the Test Editor. Then we compared the attributes of both instances. The netPremiumPm attribute we used so far, is a derived attribute for which a member variable is generated. The value of this variable is computed by explicitly calling a method (in this case inputHomeContract.computePremium()
). This type of derived attributes can be tested just like mutable attributes.
In Faktor-IPS we may also define derived attributes that are computed "on the fly" each time the getter method gets called. No member variable is generated for these attributes. Thus, they constitute a special case for test implementations because in our Expected instance, we don’t have any member variable that can possibly be used in a comparison operation.
The home contract’s ratingDistrict is such an attribute. We will now create a new test case type (named RatingDistrictTest) in order to verify the rating district computation based on the zip code.
We define the attributes zipCode of the type HomeContract as input parameters and we enable the Requires Product Component checkbox for HomeContract. Now we attach a new attribute called exptectedDistrict to the type HomeContract by clicking the Add button.
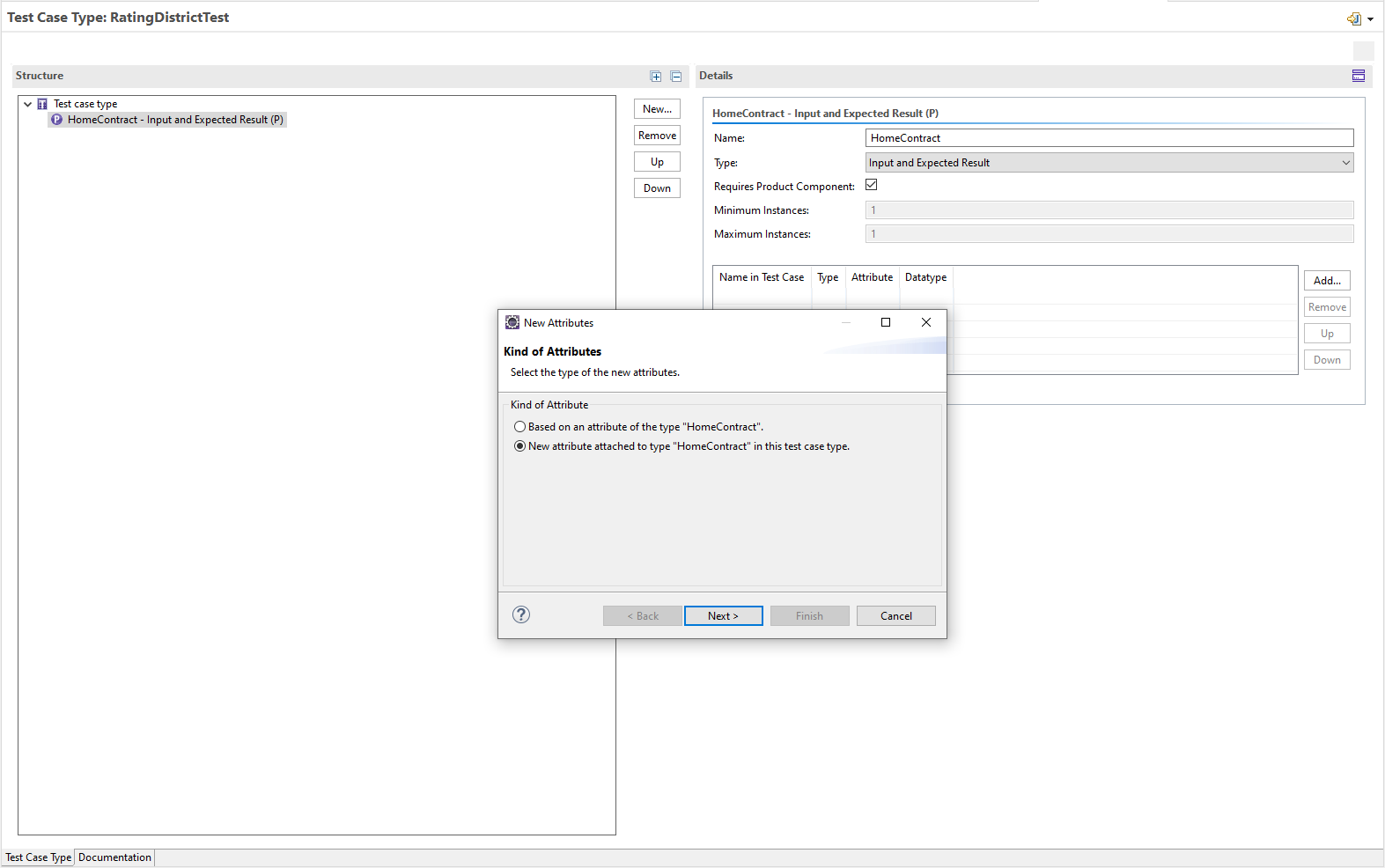
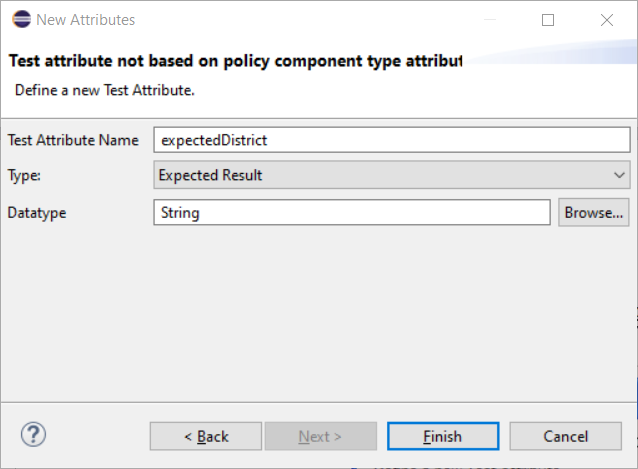
Now save the test case type and open the Java class RatingDistrictTest
that is generated for it. In the Java source code you can access the value of "attached" attributes via getExtensionAttributeValue(String attrName)
. Instead of hard coding the attribute name you should use the constant that is generated for it. The following code section shows the details.
public class RatingDistrictTest extends IpsTestCase2 {
/**
* @generated
*/
public static final String TESTATTR_HOME_CONTRACT_EXPECTED_DISTRICT = "expectedDistrict";
//...
/**
* Executes the business logic under test.
*
* @restrainedmodifiable
*/
@Override
@IpsGenerated
public void executeBusinessLogic() {
// begin-user-code
// nothing to do (the logic to be tested is run by calling the getter)
// end-user-code
}
/**
* Executes the asserts that compare actual with expected result.
*
* @restrainedmodifiable
*/
@Override
@IpsGenerated
public void executeAsserts(IpsTestResult result) {
// begin-user-code
String expectedDistrict = (String)getExtensionAttributeValue(
expectedHomeContract, TESTATTR_HOME_CONTRACT_EXPECTED_DISTRICT);
String computedDistrict = inputHomeContract.getRatingDistrict();
assertEquals(expectedDistrict, computedDistrict, result,
"HomeContract#0", TESTATTR_HOME_CONTRACT_EXPECTED_DISTRICT);
// end-user-code
}
//...
}
In order to verify this, we create a test case (RatingDistrictTest_1) based on the new test case type and populate the test data for ZipCode. In addition, we assign a home product (e.g., HC-Compact 2021-12) to the contract. According to the rating district table we expect the district VI for zip code 63066:

After running the test, a green bar confirms that our test case is correct.